5.3. LibAVStream¶
5.3.1. Introduction¶
LibAVStream is a helper library for streaming video and geometry data between a server and client. A user of this library constructs an avs::Pipeline instance, and configures the pipeline with avs::PipelineNode subclass instances. Nodes can receive data from other nodes, process it, and pass the processed data on to other nodes.
A pipeline operates on a single thread, while queues allow threads to exchange data. For example, the server’s Network pipeline connects several avs::Queue instances to a single avs::NetworkSink. For example, the video encoding pipeline links an avs::Surface that receives raw video frames, to an avs::Encoder, and finally to a queue. On another thread, the same queue in the network pipeline passes the data to the avs::NetworkSink.
LibAVStream uses Enet for UDP datagram control. It uses SRT and EFP over UDP for data stream reliability and deliverability.
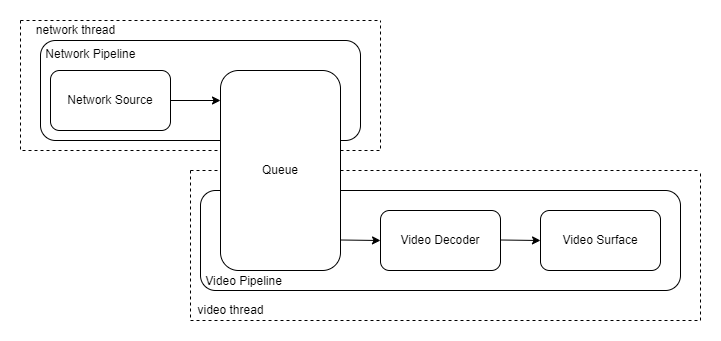
Fig. 5.1 Two pipelines operating on separate threads are connected using an avs::Queue.¶
5.3.2. Namespace¶
-
namespace avs¶
Typedefs
-
typedef uint64_t uid¶
-
typedef uint16_t InputId¶
An input identifier, used between client and server to denote a specific input.
-
typedef void (*MessageHandlerFunc)(LogSeverity severity, const char *msg, void *userData)¶
Message handler function prototype.
- Param severity:
Message severity class.
- Param msg:
Null-terminated string containing the message.
- Param userData:
Custom user data pointer.
-
typedef std::function<void(const uint8_t *buffer, size_t bufferSize)> HTTPCallbackFn¶
-
typedef void *LibraryHandle¶
-
typedef void *ProcAddress¶
-
typedef struct timespec Timestamp¶
-
typedef PlatformPOSIX Platform¶
Enums
-
enum class AudioEncoderBackend¶
Audio encoder backend type.
Values:
-
enumerator Any¶
Any backend (auto-detect during configuration).
-
enumerator Custom¶
Custom external backend.
-
enumerator Any¶
-
enum class LogSeverity¶
Log severity class.
Values:
-
enumerator Never¶
-
enumerator Debug¶
-
enumerator Info¶
-
enumerator Warning¶
-
enumerator Error¶
-
enumerator Critical¶
-
enumerator Num_LogSeverity¶
-
enumerator Never¶
-
enum class DeviceType¶
Graphics API device handle type.
Values:
-
enumerator Invalid¶
Invalid (null) device.
-
enumerator Direct3D11¶
Direct3D 11 device.
-
enumerator Direct3D12¶
Direct3D 12 device
-
enumerator OpenGL¶
OpenGL device (implicit: guarantees that OpenGL context is current in calling thread).
-
enumerator Vulkan¶
Vulkan device
-
enumerator Invalid¶
-
enum class VideoCodec : uint8_t¶
Video codec.
Values:
-
enumerator Any¶
-
enumerator Invalid¶
-
enumerator H264¶
H264
-
enumerator HEVC¶
HEVC (H265)
-
enumerator Any¶
-
enum class VideoPreset¶
Video encoding preset.
Values:
-
enumerator Default¶
Default encoder preset.
-
enumerator HighPerformance¶
High performance preset (potentially faster).
-
enumerator HighQuality¶
High quality preset (potentially slower).
-
enumerator Default¶
-
enum class VideoPayloadType : uint8_t¶
Video payload type.
Values:
-
enumerator FirstVCL¶
Video Coding Layer unit (first VCL in an access unit).
-
enumerator VCL¶
Video Coding Layer unit (any subsequent in each access unit).
-
enumerator VPS¶
Video Parameter Set (HEVC only)
-
enumerator SPS¶
Sequence Parameter Set
-
enumerator PPS¶
Picture Parameter Set
-
enumerator ALE¶
Custom name. NAL unit with alpha layer encoding metadata (HEVC only).
-
enumerator OtherNALUnit¶
Other NAL unit.
-
enumerator AccessUnit¶
Entire access unit (possibly multiple NAL units).
-
enumerator FirstVCL¶
-
enum class NetworkDataType : uint8_t¶
Values:
-
enumerator H264¶
-
enumerator HEVC¶
-
enumerator Framed¶
-
enumerator Generic¶
-
enumerator H264¶
-
enum class GeometryPayloadType : uint8_t¶
Values:
-
enumerator Invalid¶
-
enumerator Mesh¶
-
enumerator Material¶
-
enumerator MaterialInstance¶
-
enumerator Texture¶
-
enumerator Animation¶
-
enumerator Node¶
-
enumerator Skeleton¶
-
enumerator FontAtlas¶
-
enumerator TextCanvas¶
-
enumerator Invalid¶
-
enum class NodeDataType : uint8_t¶
Values:
-
enumerator Invalid¶
-
enumerator None¶
-
enumerator Mesh¶
-
enumerator Light¶
-
enumerator TextCanvas¶
-
enumerator SubScene¶
-
enumerator Skeleton¶
-
enumerator Link¶
-
enumerator Invalid¶
-
enum class FilePayloadType : uint8_t¶
Values:
-
enumerator Invalid¶
-
enumerator Texture¶
-
enumerator Mesh¶
-
enumerator Material¶
-
enumerator Invalid¶
-
enum class StreamingConnectionState : uint8_t¶
Values:
-
enumerator UNINITIALIZED¶
-
enumerator NEW_UNCONNECTED¶
-
enumerator CONNECTING¶
-
enumerator CONNECTED¶
-
enumerator DISCONNECTED¶
-
enumerator FAILED¶
-
enumerator CLOSED¶
-
enumerator ERROR_STATE¶
-
enumerator UNINITIALIZED¶
-
enum class InputType : uint8_t¶
What type of input to send, and when.
Values:
-
enumerator Invalid¶
-
enumerator IsEvent¶
-
enumerator IsReleaseEvent¶
-
enumerator IsInteger¶
-
enumerator IsFloat¶
-
enumerator IntegerState¶
-
enumerator FloatState¶
-
enumerator IntegerEvent¶
-
enumerator ReleaseEvent¶
-
enumerator FloatEvent¶
-
enumerator Invalid¶
-
enum class AxesStandard : uint8_t¶
A model for how 3D space is mapped to X, Y and Z axes. A server may use one standard internally, while clients may use others. A server must be capable of supporting clients in at least EngineeringStyle and GlStyle.
Values:
-
enumerator NotInitialized¶
-
enumerator RightHanded¶
-
enumerator LeftHanded¶
-
enumerator YVertical¶
-
enumerator ZVertical¶
-
enumerator EngineeringStyle¶
-
enumerator GlStyle¶
-
enumerator UnrealStyle¶
-
enumerator UnityStyle¶
-
enumerator NotInitialized¶
-
enum class DecoderBackend¶
Decoder backend type.
Values:
-
enumerator Any¶
Any backend (auto-detect during configuration).
-
enumerator Custom¶
Custom external backend.
-
enumerator NVIDIA¶
NVIDIA CUVID backend.
-
enumerator AMD¶
AMD CUVID backend.
-
enumerator Any¶
-
enum class DecodeFrequency¶
Payload decode frequency.
Defines at what granularity video payload is being passed to the underlying hardware decoder. Different decoders may have different requirements as to the format of input they accept.
Values:
-
enumerator AccessUnit¶
Decode every complete access unit (at VCL boundaries).
-
enumerator NALUnit¶
Decode every NAL unit.
-
enumerator AccessUnit¶
-
enum class DecoderStatus : uint32_t¶
Video decoder status.
Specifies the status of the video decoder at certain step in the video decoding pipeline.
Values:
Decoder has not been set up.
-
enumerator QueuingVideoStreamBuffer¶
Decoder is storing the single received buffer for accumulation and processing.
-
enumerator AccumulatingVideoStreamBuffers¶
Decoder is collecting multiple single buffers to assemble enough data for a frame.
-
enumerator DecodingVideoStream¶
Hardware or software accelerated decoding of the video stream data.
-
enumerator ProcessingOutputFrameFromDecoder¶
Decoder is processing the output frame for use in graphics APIs.
-
enumerator FrameAvailable¶
Decoded video frame is available for use in graphics APIs.
-
enum class DecoderStatusNames¶
Video decoder status names.
For use with magic_enum
Values:
-
enumerator DecoderAvailable¶
-
enumerator ReceivingVideoStream¶
-
enumerator QueuingVideoStreamBuffer¶
-
enumerator AccumulatingVideoStreamBuffers¶
-
enumerator PassingVideoStreamToDecoder¶
-
enumerator DecodingVideoStream¶
-
enumerator ProcessingOutputFrameFromDecoder¶
-
enumerator FrameAvailable¶
-
enumerator DecoderAvailable¶
-
enum class EncoderBackend¶
Encoder backend type.
Values:
-
enumerator Any¶
Any backend (auto-detect during configuration).
-
enumerator Custom¶
Custom external backend.
-
enumerator NVIDIA¶
NVIDIA NVENC backend.
-
enumerator Any¶
-
enum class RateControlMode¶
Values:
-
enumerator RC_CONSTQP¶
Constant QP mode
-
enumerator RC_VBR¶
Variable bitrate mode
-
enumerator RC_CBR¶
Constant bitrate mode
-
enumerator RC_CONSTQP¶
-
enum class FileAccess¶
Values:
-
enumerator None¶
Unconfigured.
-
enumerator Read¶
Read only access.
-
enumerator Write¶
Write only access.
-
enumerator None¶
-
enum class SamplerFilter : uint32_t¶
Values:
-
enumerator NEAREST¶
-
enumerator LINEAR¶
-
enumerator NEAREST_MIPMAP_NEAREST¶
-
enumerator LINEAR_MIPMAP_NEAREST¶
-
enumerator NEAREST_MIPMAP_LINEAR¶
-
enumerator LINEAR_MIPMAP_LINEAR¶
-
enumerator NEAREST¶
-
enum class SamplerWrap : uint32_t¶
Values:
-
enumerator REPEAT¶
-
enumerator CLAMP_TO_EDGE¶
-
enumerator CLAMP_TO_BORDER¶
-
enumerator MIRRORED_REPEAT¶
-
enumerator MIRROR_CLAMP_TO_EDGE¶
-
enumerator REPEAT¶
-
enum class TextureFormat : uint32_t¶
Values:
-
enumerator INVALID¶
-
enumerator G8¶
-
enumerator BGRA8¶
-
enumerator BGRE8¶
-
enumerator RGBA16¶
-
enumerator RGBA16F¶
-
enumerator RGBA8¶
-
enumerator RGBE8¶
-
enumerator D16F¶
-
enumerator D24F¶
-
enumerator D32F¶
-
enumerator RGBA32F¶
-
enumerator RGB8¶
-
enumerator MAX¶
-
enumerator INVALID¶
-
enum class TextureCompression : uint32_t¶
Values:
-
enumerator UNCOMPRESSED¶
-
enumerator BASIS_COMPRESSED¶
-
enumerator PNG¶
-
enumerator KTX¶
-
enumerator UNCOMPRESSED¶
-
enum class RoughnessMode : uint16_t¶
Values:
-
enumerator CONSTANT¶
-
enumerator ROUGHNESS¶
-
enumerator SMOOTHNESS¶
-
enumerator CONSTANT¶
-
enum class MaterialMode : uint8_t¶
Values:
-
enumerator UNKNOWNMODE¶
-
enumerator OPAQUE_MATERIAL¶
-
enumerator TRANSPARENT_MATERIAL¶
-
enumerator UNKNOWNMODE¶
-
enum class PrimitiveMode : uint32_t¶
Values:
-
enumerator POINTS¶
-
enumerator LINES¶
-
enumerator TRIANGLES¶
-
enumerator LINE_STRIP¶
-
enumerator TRIANGLE_STRIP¶
-
enumerator POINTS¶
-
enum class AttributeSemantic : uint32_t¶
The standard glTF attribute semantics.
Values:
-
enumerator POSITION¶
-
enumerator NORMAL¶
-
enumerator TANGENT¶
-
enumerator TEXCOORD_0¶
-
enumerator TEXCOORD_1¶
-
enumerator COLOR_0¶
-
enumerator JOINTS_0¶
-
enumerator WEIGHTS_0¶
-
enumerator TANGENTNORMALXZ¶
-
enumerator COUNT¶
-
enumerator POSITION¶
-
enum class MeshCompressionType : uint8_t¶
Values:
-
enumerator NONE¶
-
enumerator DRACO¶
-
enumerator DRACO_VERSIONED¶
-
enumerator NONE¶
-
enum class StreamParserType¶
Bitstream parser type
Values:
-
enumerator AVC_AnnexB¶
-
enumerator Geometry¶
-
enumerator Audio¶
-
enumerator Custom¶
-
enumerator None¶
-
enumerator Default¶
-
enumerator AVC_AnnexB¶
-
enum class SurfaceFormat¶
Surface format.
Values:
-
enumerator Unknown¶
Unknown or invalid format.
-
enumerator ARGB¶
8-bits per channel ARGB format.
-
enumerator ABGR¶
8-bits per channel ABGR format.
-
enumerator NV12¶
NV12 format (YCrCb color encoding).
-
enumerator R16¶
16-bits single channel format.
-
enumerator ARGB10¶
10-bits per color channels, 2 bits for alpha. ARGB10 format.
-
enumerator ABGR10¶
10-bits per color channels, 2 bits for alpha. ABGR10 format.
-
enumerator ARGB16¶
16-bits per channel ARGB16 format.
-
enumerator ABGR16¶
16-bits per channel ABGR16 format.
-
enumerator Unknown¶
Functions
- AVSTREAM_API uid GenerateUid ()
- AVSTREAM_API void ClaimUidRange (avs::uid last)
-
inline const char *stringOf(GeometryPayloadType t)¶
-
inline const char *stringOf(StreamingConnectionState state)¶
-
inline AxesStandard operator|(const AxesStandard &a, const AxesStandard &b)¶
-
inline AxesStandard operator&(const AxesStandard &a, const AxesStandard &b)¶
-
template<>
inline bool verify_values(const float &t1, const float &t2)¶
-
template<>
inline bool verify_values(const vec2 &t1, const vec2 &t2)¶
-
template<>
inline bool verify_values(const vec3 &t1, const vec3 &t2)¶
-
template<>
inline bool verify_values(const vec4 &t1, const vec4 &t2)¶
-
static inline std::string convertToByteString(std::wstring wideString)¶
-
static inline std::string convertToByteString(const char *txt)¶
-
template<typename istream>
istream &operator>>(istream &in, RoughnessMode &obj)¶
-
template<>
inline bool verify_values(const TextureAccessor &t1, const TextureAccessor &t2)¶
-
template<>
inline bool verify_values(const PBRMetallicRoughness &t1, const PBRMetallicRoughness &t2)¶
-
inline bool CompareMemory(const void *a, const void *b, size_t s)¶
- void AVSTREAM_API ConvertTransform (AxesStandard fromStandard, AxesStandard toStandard, Transform &transform)
- void AVSTREAM_API ConvertRotation (AxesStandard fromStandard, AxesStandard toStandard, vec4 &rotation)
- void AVSTREAM_API ConvertPosition (AxesStandard fromStandard, AxesStandard toStandard, vec3 &position)
- void AVSTREAM_API ConvertScale (AxesStandard fromStandard, AxesStandard toStandard, vec3 &scale)
- int8_t AVSTREAM_API ConvertAxis (AxesStandard fromStandard, AxesStandard toStandard, int8_t axis)
-
struct Accessor¶
-
struct Attribute¶
-
struct AudioConfig¶
-
class AudioDecoder : public avs::PipelineNode¶
- #include <audiodecoder.h>
Audio decoder node
[input-active, output-active, 1/1]
Reads packets of encoded audio and outputs decoded data to an Audio Target.
-
class AudioEncoder : public avs::PipelineNode¶
- #include <audioencoder.h>
Audio encoder node
[input-active, output-active, 1/1]
Encodes audio
Compatible outputs: Any node implementing IOInterface.
-
class AudioEncoderBackendInterface : public avs::UseInternalAllocator¶
- #include <audio_interface.h>
Audio encoder backend interface.
Subclassed by teleport::server::AudioEncoder
-
struct AudioEncoderParams¶
- #include <audio_interface.h>
Audio encoder parameters.
-
class AudioParserInterface¶
- #include <audio_interface.h>
Audio parser interface.
-
class AudioTarget : public avs::PipelineNode, public avs::AudioTargetInterface¶
- #include <audiotarget.h>
A class to receive and process streamed audio
-
class AudioTargetBackendInterface : public avs::UseInternalAllocator¶
- #include <audio_interface.h>
Audio target backend interface.
-
class AudioTargetInterface¶
- #include <interfaces.hpp>
Audio target interface.
Nodes implementing this interface can act as data sinks for the purpose of decoding and playing audio.
Subclassed by avs::AudioTarget
-
class Buffer : public avs::PipelineNode, public avs::IOInterface¶
- #include <buffer.hpp>
Ring buffer node
[passive, 1/1]
A thread-safe, nonblocking, producer-consumer ring buffer with fixed capacity.
-
struct BufferView¶
- #include <mesh_interface.hpp>
A view into a buffer. Could either be a contiguous subset of the data, or a stride-view skipping elements.
-
class ClientServerMessageStack : public avs::GenericEncoderBackendInterface¶
-
struct CompressedMesh¶
-
struct CompressedSubMesh¶
-
class Context¶
- #include <context.hpp>
Global library context.
Context object managing application-global state. A single instance of this class must be instantiated by the application before accessing any other library functionality.
-
class CreateSessionDescriptionObserver : public webrtc::CreateSessionDescriptionObserver¶
-
class DataChannelObserver : public webrtc::DataChannelObserver¶
-
class Decoder : public avs::PipelineNode¶
- #include <decoder.hpp>
Video decoder node
[input-active, output-active, 1/1]
Reads packets of encoded video stream and outputs decoded frames to a surface.
Compatible inputs: Any node implementing PacketInterface.
Compatible outputs: Any node implementing SurfaceInterface.
-
class DecoderBackendInterface : public avs::UseInternalAllocator¶
- #include <dec_interface.hpp>
Common decoder backend interface.
Decoder backend is responsible for decoding compressed video data using a particular hardware decoder. Decoded video frames are outputted to a registered surface.
Subclassed by teleport::android::VideoDecoderBackend, teleport::clientrender::VideoDecoderBackend
-
struct DecoderParams¶
- #include <dec_interface.hpp>
Video decoder parameters.
-
struct DecoderState¶
-
struct DecoderStats¶
-
struct DeviceHandle¶
- #include <common.hpp>
Graphics API device handle.
-
struct DisplayInfo
- #include <common.hpp>
Information on the resolution of a client’s display.
-
struct EncodeCapabilities¶
-
class Encoder : public avs::PipelineNode¶
- #include <encoder.hpp>
Video encoder node
[input-active, output-active, 1/1]
Encodes video frames from input surface and outputs compressed bitstream.
Compatible inputs : Any node implementing SurfaceInterface.
Compatible outputs: Any node implementing IOInterface.
-
class EncoderBackendInterface : public avs::UseInternalAllocator¶
- #include <enc_interface.hpp>
Common encoder backend interface.
Encoder backend is responsible for encoding pictures using a particular hardware encoder.
-
struct EncoderParams¶
- #include <enc_interface.hpp>
Video encoder parameters.
-
class File : public avs::PipelineNode, public avs::IOInterface, public avs::PacketInterface¶
- #include <file.hpp>
File node
[passive, 1/1]
File node provides a way to read or write from binary files and thus can act either as a data sink or data source depending on configured FileAccess mode.
-
struct FilePayloadInfo : public avs::PayloadInfo¶
-
class Forwarder : public avs::PipelineNode¶
- #include <forwarder.hpp>
Forwarder node
[input-active, output-active, N/M]
Forwarder node reads data from its inputs and passes it to its outputs without modification. It is most useful as a proxy node if one wishes to link two passive nodes within a pipeline.
Compatible inputs: Any node implementing either PacketInterface or IOInterface.
Compatible outputs: Any node implementing either PacketInterface or IOInterface.
If an input or an output of a Forwarder node implements both PacketInterface and IOInterface then PacketInterface is used by the forwarder node to read or write data.
-
class GenericDecoder : public avs::PipelineNode¶
- #include <genericdecoder.h>
Generic decoder node
[input-active, output-active, 1/1]
Reads generic packets outputs to a Generic Target.
-
class GenericEncoder : public avs::PipelineNode¶
- #include <genericencoder.h>
Video encoder node
[input-active, output-active, 1/1]
Encodes video frames from input surface and outputs compressed bitstream.
Compatible inputs : Any node implementing SurfaceInterface.
Compatible outputs: Any node implementing IOInterface.
-
class GenericEncoderBackendInterface¶
Subclassed by avs::ClientServerMessageStack
-
class GenericTargetInterface¶
Subclassed by teleport::client::SessionClient, teleport::server::ClientMessaging
-
struct GeometryBuffer¶
- #include <mesh_interface.hpp>
A buffer of arbitrary binary data, which should not be freed.
-
class GeometryDecoder : public avs::PipelineNode¶
- #include <geometrydecoder.hpp>
Geometry decoder node
[input-active, output-active, 1/1]
Reads packets of encoded geometry and outputs decoded data to a Geometry Target.
-
class GeometryDecoderBackendInterface : public avs::UseInternalAllocator¶
- #include <mesh_interface.hpp>
A Geometry decoder backend converts a.
Subclassed by teleport::clientrender::GeometryDecoder
-
class GeometryEncoder : public avs::PipelineNode¶
- #include <geometryencoder.hpp>
Video encoder node
[input-active, output-active, 1/1]
Encodes video frames from input surface and outputs compressed bitstream.
Compatible inputs : Any node implementing SurfaceInterface.
Compatible outputs: Any node implementing IOInterface.
-
class GeometryEncoderBackendInterface : public avs::UseInternalAllocator¶
- #include <mesh_interface.hpp>
A Geometry decoder backend provides geometry packets to a geometryencoder.
Subclassed by teleport::server::GeometryEncoder
-
class GeometryParserInterface¶
-
class GeometryRequesterBackendInterface : public avs::UseInternalAllocator¶
- #include <mesh_interface.hpp>
This tells the Geometry Source node what it wants from the Geometry Source backend so the node can acquire the data to be sent for encoding.
Subclassed by teleport::server::GeometryStreamingService
-
class GeometrySource : public avs::PipelineNode, public avs::GeometrySourceInterface¶
- #include <mesh.hpp>
Mesh node
[passive, 1/1]
Provides access to geometry source data for other nodes in the pipeline.
-
class GeometrySourceInterface¶
- #include <interfaces.hpp>
Mesh interface.
Nodes implementing this interface can act as data sources/sinks for the purpose of providing access to a mesh.
Subclassed by avs::GeometrySource
-
class GeometryTarget : public avs::PipelineNode, public avs::GeometryTargetInterface¶
- #include <mesh.hpp>
Mesh node
[passive, 1/1]
Provides access to geometry source data for other nodes in the pipeline.
-
class GeometryTargetBackendInterface : public avs::UseInternalAllocator¶
- #include <mesh_interface.hpp>
Common mesh decoder backend interface.
Mesh backend receives data payloads and will convert them to geometry.
Subclassed by teleport::clientrender::ResourceCreator
-
class GeometryTargetInterface¶
- #include <interfaces.hpp>
Geometry target interface.
Nodes implementing this interface can act as data sinks for the purpose of building geometry.
Subclassed by avs::GeometryTarget
-
struct guid¶
-
struct HTTPPayloadRequest¶
-
class HTTPUtil¶
- #include <httputil.hpp>
Utility for making HTTP/HTTPS requests.
-
struct HTTPUtilConfig¶
-
struct InputEventAnalogue¶
- #include <common_input.h>
Input events that can be normalised between two values; e.g. how pressed a trigger is.
-
struct InputEventBinary¶
- #include <common_input.h>
Input events that can only be in two states; e.g. button pressed or not.
-
struct InputEventMotion¶
- #include <common_input.h>
Input events that represent the motion in two directions; e.g. a stick on a controller.
-
class IOInterface¶
- #include <interfaces.hpp>
General (stream of bytes) I/O node interface.
Nodes implementing this interface can act as data sources and/or sinks for the purpose of arbitrary reads and/or writes.
Subclassed by avs::Buffer, avs::File, avs::NullSink, avs::Packetizer, avs::Queue, avs::SingleQueue
-
struct LightNodeResources¶
-
struct Link¶
-
struct Mat4x4¶
-
struct Material¶
-
class MaterialExtension¶
Subclassed by avs::SimpleGrassWindExtension
-
struct MaterialResources¶
-
struct Mesh¶
-
struct MeshCreate¶
-
struct MeshElementCreate¶
- #include <mesh_interface.hpp>
Structure to pass to GeometryTargetBackendInterface
-
struct MeshNodeResources¶
-
class NetworkSink : public avs::PipelineNode¶
Subclassed by avs::NullNetworkSink, avs::WebRtcNetworkSink
-
struct NetworkSinkCounters¶
- #include <networksink.h>
Network sink counters.
-
struct NetworkSinkParams¶
- #include <networksink.h>
Network sink parameters.
-
struct NetworkSinkStream¶
- #include <networksink.h>
Network sink stream data.
-
class NetworkSource : public avs::PipelineNode¶
- #include <networksource.h>
Network source node
[passive, 0/1]
Receives video stream from a remote UDP endpoint.
Subclassed by avs::WebRtcNetworkSource
-
struct NetworkSourceCounters¶
- #include <networksource.h>
Network source counters.
-
struct NetworkSourceParams¶
- #include <networksource.h>
Network source parameters.
-
struct NetworkSourceStream¶
- #include <networksource.h>
Network source stream data.
-
struct Node¶
-
struct NodeRenderState¶
-
class NullNetworkSink : public avs::NetworkSink¶
-
class NullSink : public avs::PipelineNode, public avs::IOInterface, public avs::PacketInterface¶
- #include <nullsink.hpp>
Null sink node
[passive, N/0]
Silently discards all data written to it.
-
class PacketInterface¶
- #include <interfaces.hpp>
Packet I/O node interface.
Nodes implementing this interface can act as data sources and/or sinks for the purpose of packet reads and/or writes. Packet reads (writes) operate on atomic chunks of data - packets cannot be partially read or written.
Subclassed by avs::File, avs::NullSink
-
class Packetizer : public avs::PipelineNode, public avs::IOInterface¶
- #include <packetizer.hpp>
Bitstream packetizer node
[output-active, 1/M]
Accepts bitstream as input and broadcasts discrete packets to all its outputs. Expected bitstream format and output packets payload is defined by the selected stream parser.
When used with AVC_AnnexB parser it accepts AVC Annex B bitstream input and outputs individual NAL units.
Compatible outputs: Any node implementing PacketInterface.
-
struct PayloadInfo¶
Subclassed by avs::FilePayloadInfo, avs::StreamPayloadInfo
-
struct PBRMetallicRoughness¶
-
class PeerConnectionObserver : public webrtc::PeerConnectionObserver¶
-
class Pipeline¶
- #include <pipeline.hpp>
A pipeline.
A pipeline is a collection of nodes linked together in a serial manner such that each node produces output for the next node. A pipeline does not take ownership of its nodes; a single node can be a member of more than one pipeline.
It is recommended to create no more than a single pipeline per application thread and link pipelines via shared Queue or Buffer nodes to ensure thread safety.
-
class PipelineNode¶
- #include <node.hpp>
Abstract processing node.
Processing nodes are fundamental building blocks of pipelines. Each node can have N input slots, and M output slots (N,M >= 0), and can do some amount of work during pipeline processing.
Nodes can be classified as “input-active” and/or “output-active”, or “passive”.
Input-Active nodes read data from their inputs during processing.
Output-Active nodes write data to their outputs during processing.
Passive nodes usually don’t do any processing and just act as data sources & sinks to other, active, nodes.
Being aware of this classification is very important in correctly constructing pipelines.
Subclassed by avs::AudioDecoder, avs::AudioEncoder, avs::AudioTarget, avs::Buffer, avs::Decoder, avs::Encoder, avs::File, avs::Forwarder, avs::GenericDecoder, avs::GenericEncoder, avs::GeometryDecoder, avs::GeometryEncoder, avs::GeometrySource, avs::GeometryTarget, avs::NetworkSink, avs::NetworkSource, avs::NullSink, avs::Packetizer, avs::Queue, avs::SingleQueue, avs::Surface, avs::TagDataDecoder
-
class PlatformPOSIX¶
-
class PlatformWindows¶
-
struct Pose¶
-
struct PoseDynamic¶
-
struct PrimitiveArray¶
-
class Queue : public avs::PipelineNode, public avs::IOInterface¶
- #include <queue.hpp>
Queue node
[passive, 1/1]
A thread-safe, nonblocking, producer-consumer queue of byte buffers.
Sharing an instance of this node is the recommended way to link two pipelines running on different threads.
-
struct RenderingFeatures¶
- #include <common.hpp>
Features supported by a client.
-
struct Sampler¶
-
template<typename T>
class Serial¶
-
class SetSessionDescriptionObserver : public webrtc::SetSessionDescriptionObserver¶
-
struct SetupMessage¶
- #include <networksink.h>
A message sent by the reliable (e.g. Websockets) channel.
-
class SimpleGrassWindExtension : public avs::MaterialExtension¶
-
class SingleQueue : public avs::PipelineNode, public avs::IOInterface¶
- #include <singlequeue.h>
Queue node
[passive, 1/1]
A thread-safe, nonblocking, pseudo-queue of either one, or zero byte buffers. Pushing a buffer replaces the one that’s there, if any. Sharing an instance of this node is a way to link two pipelines running on different threads.
-
struct Skeleton¶
-
class StreamParserInterface : public avs::UseInternalAllocator¶
- #include <parser_interface.hpp>
Common stream parser interface.
Stream parser parses raw bitstream into logical codec packets (usually NAL units).
-
struct StreamPayloadInfo : public avs::PayloadInfo¶
-
struct StreamStatus¶
-
class Surface : public avs::PipelineNode, public avs::SurfaceInterface¶
- #include <surface.hpp>
Surface node
[passive, 1/1]
Provides access to surface for other nodes in the pipeline.
-
class SurfaceBackendInterface : public avs::UseInternalAllocator¶
- #include <surface_interface.hpp>
Common surface backend interface.
Surface backend abstracts graphics API specific hardware-accelerated surface handle.
Subclassed by avs::SurfaceDX11, avs::SurfaceDX12, avs::SurfaceVulkan
-
class SurfaceDX11 : public avs::SurfaceBackendInterface¶
- #include <surface_dx11.hpp>
Direct3D11 texture surface.
Instance of this class holds reference on the underlying ID3D11Texture2D object.
-
class SurfaceDX12 : public avs::SurfaceBackendInterface¶
- #include <surface_dx12.hpp>
Direct3D12 texture surface.
Instance of this class holds reference on the underlying ID3D12Resource object.
-
class SurfaceInterface¶
- #include <interfaces.hpp>
Surface node interface.
Nodes implementing this interface can act as data sources/sinks for the purpose of providing access to a surface.
Subclassed by avs::Surface
-
class SurfaceVulkan : public avs::SurfaceBackendInterface¶
- #include <surface_vulkan.hpp>
Vulkan texture surface.
Note
Instance of this class holds reference on the underlying Vulkan image object.
-
struct SystemTime¶
-
class TagDataDecoder : public avs::PipelineNode¶
- #include <tagdatadecoder.hpp>
Video tag data decoder node
[input-active, 1/0]
Reads packets of encoded video tag data and outputs result to the application.
Compatible inputs: A queue of taga data.
-
struct TagDataDecoderStats¶
-
struct Texture¶
-
struct TextureAccessor¶
-
template<class T>
class ThreadSafeQueue¶
-
class Timer¶
- #include <timer.hpp>
Timer for getting the elapsed between the start time and the current time.
-
class TimerUtil¶
- #include <timer.hpp>
Timer for getting the elapsed between the start time and the current time.
-
struct Transform¶
-
class UseInternalAllocator¶
- #include <memory.hpp>
Helper class for enforcing the use of memory allocator defined within libavstream library module.
This is used to prevent memory allocation/deallocation across DLL boundary even when operator new or operator delete are called from client code.
Subclassed by avs::AudioEncoderBackendInterface, avs::AudioTargetBackendInterface, avs::DecoderBackendInterface, avs::EncoderBackendInterface, avs::GeometryDecoderBackendInterface, avs::GeometryEncoderBackendInterface, avs::GeometryRequesterBackendInterface, avs::GeometryTargetBackendInterface, avs::StreamParserInterface, avs::SurfaceBackendInterface
-
template<typename T>
struct Vec2¶
-
template<typename T>
struct Vec3¶
-
template<typename T>
struct Vec4¶
-
struct VideoConfig¶
- #include <common.hpp>
Information on the configuration of a video stream.
-
class WebRtcNetworkSink : public avs::NetworkSink¶
- #include <webrtc_networksink.h>
Network sink node
[passive, 0/1]
Reads data for each stream from a corresponding avs::Queue input node , assembles the data into payloads of network packets and sends the data to the client.
If data throttling is enabled for a stream, the seding of data may be spread over time to reduce network congestion.
-
class WebRtcNetworkSource : public avs::NetworkSource¶
- #include <webrtc_networksource.h>
Network source node
[passive, 0/1]
Receives video stream from a remote UDP endpoint.
-
typedef uint64_t uid¶